Bicep Language - Beyond Basics - Parameters
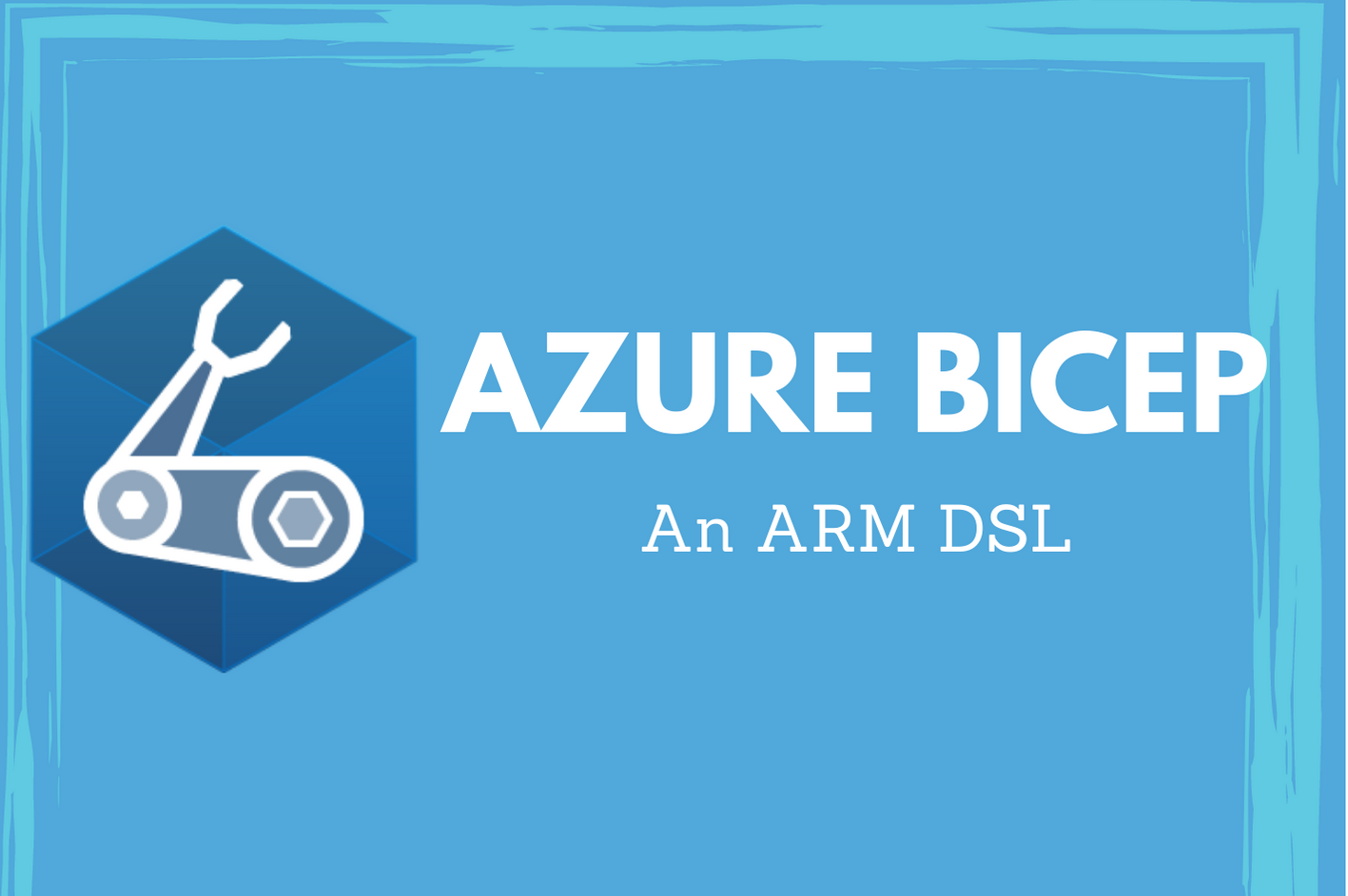
In the previous part, you learned how you can get started with Bicep language to write your first Bicep file. As you learned, resource
keyword along with resource declaration and resource properties are the minimum required in a Bicep file. In today’s article, you will see what is beyond those basics.
Parameters
One of the goals of Infrastructure as Code (IaC) practice is to create reusable and repeatable automation for deploying your infrastructure. To make this happen, you need to parameterize your infrastructure configuration definitions. In this case, your Bicep files.
Bicep language supports parameter declarations that get compiled into ARM template parameters.
At a minimum, this is how you specify a parameter declaration.
|
|
The param
keyword is what gets used to declare parameters in Bicep. The parameter-identifier
is what gets referenced when we need the value assigned to the parameter within any resource properties. The parameter-type
can be any of the supported data types in Bicep. Bicep has support for several data types like every other programming language. This includes simple types such as int
, number
, string
, bool
, null
, error
, and any
and complex types such as arrays and objects. You will learn about some of these types are you build more complex Bicep programs.
So, in its simplest form, parameter specification in a Bicep file will look like the below example.
|
|
You can assign default values to parameters. For example,
|
|
You can also assign value from an expression as a default value as well.
|
|
In Bicep, you can add parameter metadata and constraints using decorators. The general syntax for using decorated parameter declaration is as shown below.
|
|
Description Decorator
The @description
decorator is used to specify parameter description.
|
|
Value Decorator
The @minValue
and @maxValue
decorators are used to define the lower and upper bound values for an integer parameter.
|
|
Allowed Values Decorator
Similar to JSON ARM templates, Bicep language too supports constraining parameter values to a known set. This is done using @allowed
parameter decorator.
|
|
@allowed
decorator expects an array of values. This validation is case-sensitive. So, if you specify WestUs2 instead of WestUS2, Bicep build
will fail.
|
|
Length Decorator
Using the @minLength
and @maxLength
decorator, you can constrain the length of string and array data type parameter values.
|
|
Secure Decorator
The @secure
decorator specifies that the parameter is a secure string or secure object. When a parameter is marked as secure, its value does not get stored in the deployment history.
|
|
Metadata Decorator
The @metadata
decorator can be used to specify any other custom properties that describe the parameter or its significance.
|
|
You can combine multiple decorators for any given parameter in the Bicep file. Here is an example with declaration with parameter decorators.
|
|
Here is how the generated template looks once you compile this using bicep build
command.
|
|
Notice in the storageAccountName
parameter definition how the contents of metadata decorator get rolled into parameter’s metadata attribute in the JSON template.
Alright. This is all about using parameters in Bicep. You will gain some more knowledge beyond basics in the next post. Stay tuned.
Share on: